dom渲染总结
happylay 🐑 2020-12-29 代码片段dom
摘要: dom 渲染总结 时间: 2020-12-29
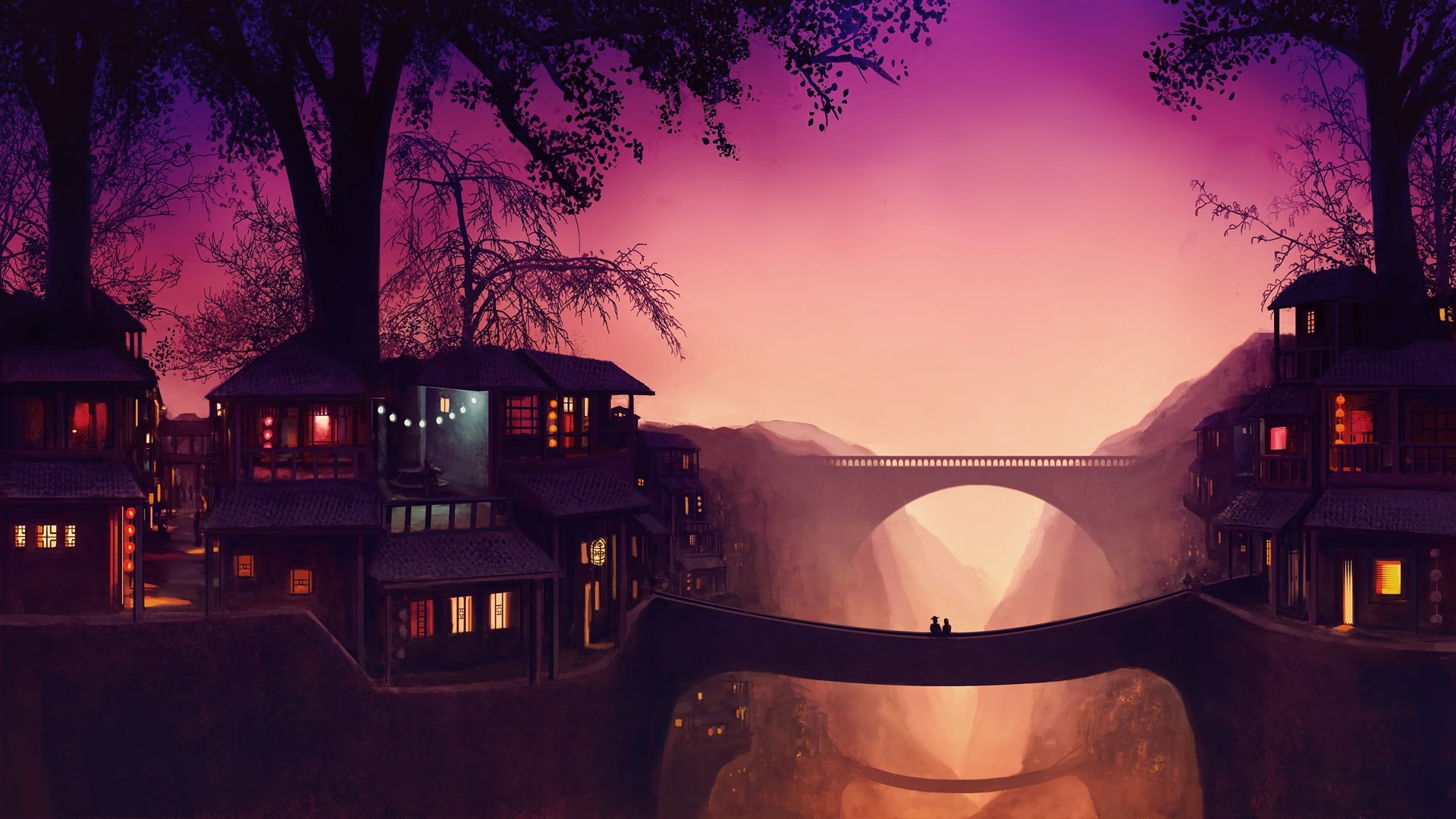
# vue dom 渲染
对对象中属性操作会被渲染,对集合操作不会被渲染,
可以使用 Vue.set(target, key, value) 或者 this.$set(target, key, value)方法修改数据
- target:要更改的数据源(可以是对象或者数组)
- key:要更改的具体数据
- value:重新赋的值
<!DOCTYPE html>
<html>
<head lang="en">
<meta charset="UTF-8" />
<title></title>
</head>
<body>
<div id="app2">
<p v-for="item in items" :key="item.id">
{{item.message}}
</p>
<p v-for="(item,index) in student.list" :key="index">
{{item}}
</p>
<pre>{{items}}</pre>
<pre>{{student}}</pre>
<button class="btn" @click="btn2Click()">动态赋值</button><br />
<button class="btn" @click="btn2errorClick()">
动态赋值(不会立即渲染)</button
><br />
<button class="btn" @click="btn2pushClick()">动态赋值(push)</button
><br />
<button class="btn" @click="btn2deleteClick()">动态赋值(splice)</button
><br />
<button class="btn" @click="btn3Click()">为data新增属性</button>
</div>
<!-- 开发环境版本,包含了有帮助的命令行警告 -->
<script
src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"
type="text/javascript"
></script>
<script>
var vm2 = new Vue({
el: "#app2",
data: {
items: [
{ message: "Test one", id: "1" },
{ message: "Test two", id: "2" },
{ message: "Test three", id: "3" },
],
student: {
id: 1,
name: "李华",
list: [
{
id: 1,
name: "张三",
},
],
},
},
methods: {
// Vue.set(target, key, value)
// target:要更改的数据源(可以是对象或者数组)
// key:要更改的具体数据
// value :重新赋的值
btn2Click: function() {
Vue.set(this.items, 0, { message: "Change Test", id: "10" });
//this.$set(this.items, 0, { message: "Change Test", id: '10' })
},
// 数据不会立即渲染
btn2errorClick: function() {
const data = [
{ message: "数据", id: "10" },
{ message: "数据", id: "20" },
{ message: "数据", id: "30" },
];
// 会渲染
this.items = data;
// 不会渲染
this.items[0] = { message: "Change Test", id: "10" };
// 备注:只要有一个数据渲染就会被同时渲染
// 会渲染
//this.student.name = '大秦赋'
// 会渲染
//this.student.list[0].name = '大秦赋'
// 会渲染
//this.student.list[0].name = { name: "大秦赋", id: '2' }
// 不会渲染
this.student.list[0] = { name: "大秦赋", id: "2" };
},
// 数据会被渲染
btn2pushClick: function() {
const l1 = { message: "Change Test", id: "10" };
this.items.push(l1);
},
btn2deleteClick: function() {
// delete 操作符会从某个对象上移除指定属性。成功删除的时候会返回 true,否则返回 false
// delete this.items[0]
// 获取删除下标序列
const index = this.items.findIndex((x) => x.id === "3");
if (index != -1) {
// 删除下标元素
this.items.splice(index, 1);
}
},
// 新增对象
btn3Click: function() {
var itemLen = this.items.length;
Vue.set(this.items, itemLen, {
message: "Test add attr",
id: itemLen,
});
},
},
});
</script>
</body>
<!-- https://www.jianshu.com/p/e6e8c45e7fd6 -->
</html>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115