vue3总结
happylay 🐑 2020-12-31 代码片段vue3
摘要: vue3 总结 时间: 2020-12-23
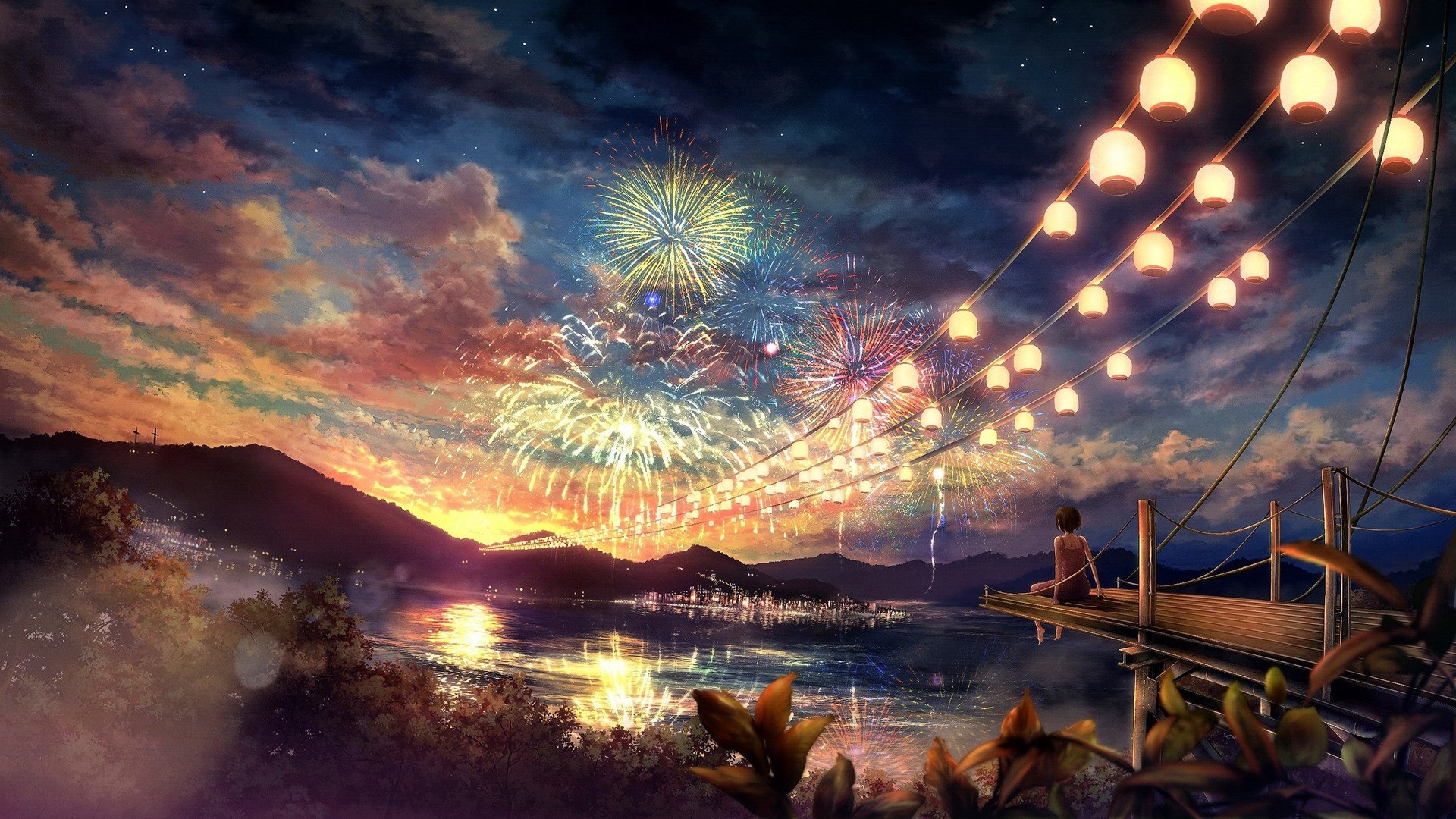
# 模板
<template>
<div></div>
</template>
<script lang="ts">
import { defineComponent, ref, toRefs, reactive, computed } from 'vue'
export default defineComponent({
name: 'happylay',
props: [],
components: {},
setup(props, context) {
// 常用写法:setup(props, { attrs, slots, emit }) {
console.log(props, context)
function emitEvent() {
context.emit('event', '注册事件')
}
const data = ref('基本类型数据,对象和数组会自动转为reactive代理对象')
const obj = reactive({
id: 1,
name: '对象',
msg: '递归深度响应式'
})
const computedValue = computed(() => {
return '计算属性' + data.value
})
const computedMethods = computed({
get() {
return
},
set(val) {
console.log('计算属性', val)
return
}
})
const getValue = () => {
console.log('获取数据(用.value获取属性值)', data.value)
}
return {
data,
obj,
...toRefs(obj), // toRefs把响应式对象转换成普通对象,普通对象的每个属性都为ref
emitEvent,
getValue,
computedValue,
computedMethods
}
},
beforeCreate() {
console.log('数据初始化的生命周期回调')
},
mounted() {
console.log(this)
}
})
</script>
<style scoped lang="scss">
</style>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
复制代码2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
# ref其余用法
<template>
<div>
<h2>获取页面元素</h2>
<input type="text" ref="inputRef" />
</div>
</template>
<script lang="ts">
import { defineComponent, ref, onMounted } from 'vue'
export default defineComponent({
setup() {
console.log('初始化')
// 默认为空,页面加载完毕,组件存在后,获取文本框元素
const inputRef = ref<HTMLElement | null>(null)
// 页面加载后
onMounted(() => {
inputRef.value && inputRef.value.focus()
})
return {
inputRef
}
}
})
</script>
<style scoped lang="scss">
</style>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
复制代码2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30