typescript 总结
happylay 🐑 2020-12-31 代码片段typescript
摘要: typescript 总结 时间: 2020-12-31
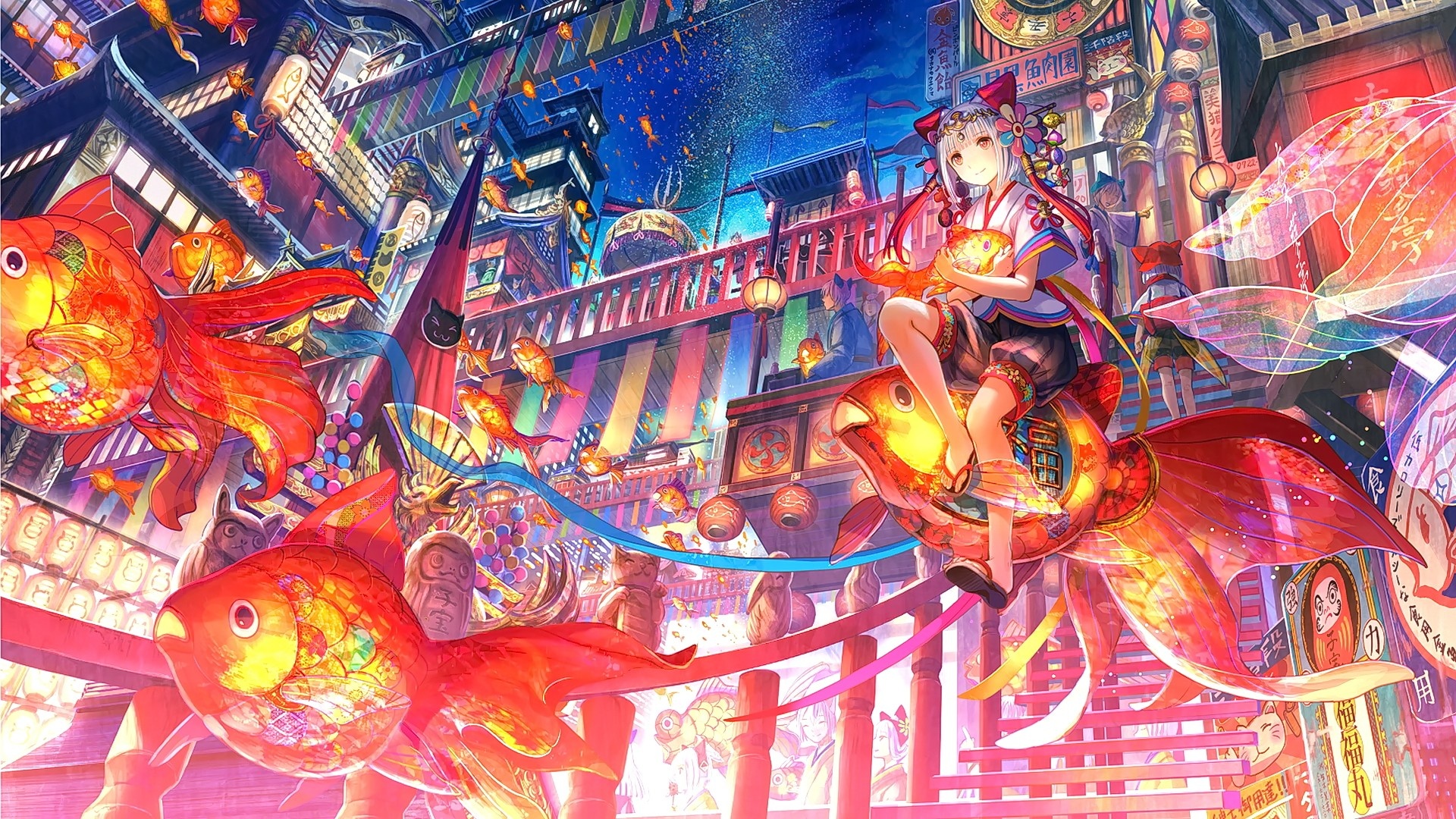
# 接口
IPerson.ts
interface IPerson {
readonly id: number
name: string
age: number
sex?: string
}
const person: IPerson = {
id: 1,
name: '嬴政',
age: 18,
// sex: '男' // 可以没有
}
console.log('%c 🍱 person: ', 'font-size:12px;background-color: #E41A6A;color:#fff;', person);
interface SearchFunc {
(source: string, subString: string): number
}
const search: SearchFunc = (source, subString) => {
return source.search(subString) == -1 ? -1 : source.search(subString) + 1
}
console.log(search('abcd', 'd'))
interface Light {
lightOn(): void
lightOff(): void
}
class Home implements Light {
lightOn(): void {
console.log('%c 🍎 开灯: ', 'font-size:12px;background-color: #2EAFB0;color:#fff;', '开灯');
}
lightOff(): void {
console.log('%c 🥘 关灯: ', 'font-size:12px;background-color: #FFDD4D;color:#fff;', '关灯');
}
}
const l1: Light = new Home()
l1.lightOn()
l1.lightOff()
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
# 元组
Tuple.ts
let t1: [string, number, boolean]
t1 = ['hi', 2020, true]
t1.push('ts')
console.log('%c 🌭 t1: ', 'font-size:12px;background-color: #B03734;color:#fff;', t1);
1
2
3
4
5
6
7
2
3
4
5
6
7
# 回调函数
CallbackFun.ts
class CallbackFun {
/**
* 结构体
*/
constructor() {
console.log('%c 🍵 初始化函数: ', 'font-size:12px;background-color: #FCA650;color:#fff;', '初始化函数');
CallbackFun.callbackTest(2020, this, this.getCallResult.bind(this))
}
/**
*
* @param arg 参数
* @param clazz 调用域
* @param callback 回调函数
*/
public static callbackTest(arg: number, clazz: any, callback: Function): void {
let result: number = ++arg;
// 回调函数
callback.call(clazz, result, '额外回调参数');
}
/**
* 回调接受函数
* @param res 结果
*/
private getCallResult(res: any, res1: any): void {
console.log('%c 🥓 回调结果:, res: ', 'font-size:12px;background-color: #FCA650;color:#fff;', "回调结果:", res);
console.log('%c 🍷 res1: ', 'font-size:12px;background-color: #EA7E5C;color:#fff;', res1);
}
}
// 调用回调函数
const f1 = new CallbackFun()
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38